More advanced delays
To make things a little easier for the script writer, you can set events to happen at a certain time with the command wait_until.
Starting off
Lets start with this, it should have everything we need to use:
/**
* Intermediate Scripting 2 - wait_until
*
* Demonstrates more advanced wait commands, specifically wait_until, which
* make it easier to write and mantain scripts.
*
* made: 2017-03-17
* by: William A. Norman
*/
// Takes a single image with red lights.
var protocol = new CameraProtocol("example_protocol")
protocol.setAnalysisID ("example")
protocol.setNumberLoops ( 1 )
protocol.setFramesPerLoop ([ 1 ])
protocol.setMeasuringLight ([ 1 ])
protocol.setSaturationFlash ([ 0 ])
protocol.setExposure ([ "50us" ])
protocol.setFrameInterval ([ "70ms" ])
// Set starting conditions
set_intensity(0)
// Take a sample imageset
run_protocol(protocol)
We're going to use the protocol to demonstrate how wait_until can effect the day.
Start at 06:00
Say we want to start this experiment at 06:00, tomorrow. But we don't want to be there that early, and we don't want to have to use any math with the wait
command. The answer is wait_until
:
// Events after this will not happen until 06:00 (real time!)
wait_until("06:00")
set_intensity(100)
run_protocol(protocol)
Go ahead and run the script now, and you'll see that it executes at the time you specified.
Gotchas
Lets take a look at the data from the run you just did.
- root
- Timeline.xml
- cam_0
- day_-1
- day_0
- cam_1
- day_-1
- day_0
- cam_2
- day_-1
- day_0
Wait, where did day_0 come from? We didn't have an EOD in that script!
It turns out the chamber cannot have events before and after the first wait_until in the same day. Because scripts frequently have starting conditions, a wait_until
, another condition, THEN the first EOD, it will implicitly switch to day 0 after the first wait_until if no EODs have been give, or if only EOD(-1) was given. It will NOT do this unless it is on day -1.
So what does this mean for you, as you write a script? If you're not using wait_until
, you don't have to worry about anything. If you are, don't call EOD until AFTER the first wait_until, or if you need EOD's before that, make sure you call it directly after the first wait_until
.
Good EOD use
Well, we don't want our data on day_-1 OR day_0.. we want it to start on day_1. So lets make it! Put this snippet directly after that wait_until
:
// Start of real data capture
EOD(1)
Reguardless of if you're using wait_until
or not, you want to declare your first EOD before your first day of real data. Days at or under 0 are generally reserved for system/debug use, and experiment setup. Don't use them on real data!
Mixing wait with wait_until
Lets add another light change, 30 seconds after hte last one:
// This will happen 30 seconds after 06:00
wait("30s")
set_intensity(200)
run_protocol(protocol)
If you run that, it will wait_until
06:00, set the intensity to 100, run the protocol, then wait
30 more seconds before setting the intensity to 200 and running the protocol again.
In effect, a wait(30min)
after a wait_until(06:00)
is exactly the same as a wait_until(06:30)
.
Gotcha again!
Try adding this to your script (but remember to delete it afterwords!)
wait("30min")
wait_until("06:28")
set_intensity(300)
run_protocol(protocol)
Compile that and lets take a look at the graph...
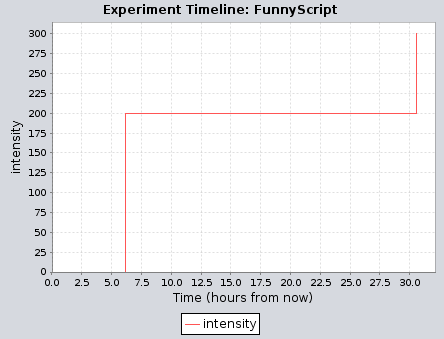
Oh my! That doesn't look like a 28 minute wait at all. That's because when the last wait_until(06:28)
hit, wait(30min)
command had already pushed it past 06:28- so the wait_until
waited until the NEXT 06:28, almost 24 hours later!
Anyway, you can delete that part of the code now.
The complete script
Okay, the complete script should now look like this:
/**
* Intermediate Scripting 2 - wait_until
*
* Demonstrates more advanced wait commands, specifically wait_until, which
* make it easier to write and mantain scripts.
*
* made: 2017-03-17
* by: William A. Norman
*/
// Takes a single image with red lights.
var protocol = new CameraProtocol("example_protocol")
protocol.setAnalysisID ("example")
protocol.setNumberLoops ( 1 )
protocol.setFramesPerLoop ([ 1 ])
protocol.setMeasuringLight ([ 1 ])
protocol.setSaturationFlash ([ 0 ])
protocol.setExposure ([ "50us" ])
protocol.setFrameInterval ([ "70ms" ])
// Set starting conditions
set_intensity(0)
// Take a sample imageset
run_protocol(protocol)
// Events after this will not happen until 06:00 (real time!)
wait_until("06:00")
// Start of real data capture
EOD(1)
set_intensity(100)
run_protocol(protocol)
// This will happen 30 seconds after 06:00
wait("30s")
set_intensity(200)
run_protocol(protocol)